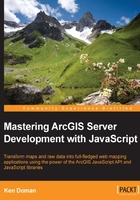
Symbols and renderers
The ArcGIS API provides some basic graphics tools to define how features look on your map. If you are creating your own custom graphics, or modifying some existing ones, this portion of the API tells you how to define their symbols. The API also provides custom renderers, which help you define what symbols are used, based on rules applied by the renderer.
Simple symbols
The ArcGIS API uses symbols to define the colors, thicknesses, and other visual features of graphics, independent of their shape. In fact, without a symbol assigned, the graphics would not show up. The API defines three simple symbols to highlight points, line, and polygons. Let's look at the Simple Symbols.
The SimpleLineSymbol
defines the symbology of line and polyline graphics added to the map. It may seem strange to start with line symbology, but the other two Simple symbols make use of a line symbol as well. The primary SimpleLineSymbol
's properties are its color, its width (in pixels), and its style. Styles include lines that are solid, dashed, dotted, and combinations of those as well.
The SimpleMarkerSymbol
defines the symbology of point and multipoint graphics on the map. The symbol can generate different shapes, depending on the style that is set. While this symbol defaults to a circle, the SimpleMarkerSymbol
can generate squares, diamonds, x's, and crosses. Other properties that can be manipulated within this symbol include the size in pixels, its outline as a SimpleLineSymbol
, and its color.
SimpeFillSymbols
define the symbology of polygon graphics on the map. The style of the SimpleFillSymbol
reflects how the inside of the shape is shown. It can be solid (completely filled in), null (completely empty), or have lines running horizontally, vertically, diagonally, or crossing. Please note that a graphic with a null-styled symbol does not respond to clicks in the center. The symbol can also be modified by a SimpleLineSymbol
outline, and if the style of the SimpleFillSymbol
is solid, its fill color can be modified as well.
Let's look a little further at the colors we'll use to define these symbols.
esri/Color
The esri/Color
module allows you to customize the colors of the graphics you add to your map. The esri/Color
module is an extension of the dojo/_base/Color
module, and adds some unique tools to blend, create, and calculate color formats in different ways. Older versions of the library used the dojo/_base/Color
module to set colors, and is still usable in the current version.
The constructor for the esri/Color
module can accept a variety of values. You can assign common color names, such as "blue" or "red". You can use hex strings (for example #FF00FF
for purple), like you would find in css colors. You can also create colors using three or four number arrays. The first three numbers in the array assign the red, green, and blue values from 0 to 255 ([0, 0, 0] is black, [255, 255, 255] is white). The fourth optional number in the array indicates the alpha, or the opacity of the color. Values in this range are from 0.0 to 1.0, with 1.0 being completely opaque, and 0.0 being completely transparent.
The esri/Color
module has some interesting enhancements on the old dojo/_base/Color
module. For instance, you can create a blend of two existing colors with the blendColors()
method. It takes two colors, and a decimal number between 0.0 and 1.0, where 0.5 is an even blend of the two colors. This could be useful, for instance, on a voting map where green represents yes, and red represents no, and voting districts could be colored by percentages of yes or no votes.
The esri/Color
modules also have ways to translate colors from one format to another. For instance, you could click on a feature, get its graphic color, and use toHex()
or toCss()
to get the color string. From there, you could apply that color to the background color of an information <div>
which is used to show the attributes of that graphic.
Picture symbols
If pictures speak to you more than colors, you can use Picture Symbols in your graphics. With these symbols, you can use picture icons and custom graphics to add interesting and memorable data points on your graph. Points and polygons can be symbolized using Picture Symbols, using the modules esri/symbols/PictureMarkerSymbol
and esri/symbols/PictureFillSymbol
respectively.
The PictureMarkerSymbol
module adds simple picture graphics for points on the map. It is typically constructed with three arguments, a URL pointing to the image file (such as .jpg
, .png
, or .gif
), and an integer width and height in pixels.
The PictureFillSymbol
module fills the content of a polygon with a repeating graphic. The PictureFillSymbol
takes four arguments. The first is a URL string pointing to an image file. The second input is a line symbol, like the esri/symbols/SimpleLineSymbol
. The final two arguments are integer width and the height of the image in pixels.
Renderers
Sometimes, you don't want to assign graphic symbols one at a time. If you know what type of graphics you're adding, and how the graphics should look ahead of time, it makes sense to assign the symbology ahead of time. That's where renderers come in. Renderers can be assigned to GraphicsLayers
as a way to assign a common style to all graphics inside them. Renderers depend on a graphics layer accepting one geometry type (like all polygons, for instance).
As the ArcGIS API has matured, it has added a number of different renderers to its library. We'll take a look at three common ones: the SimpleRenderer
, the UniqueValueRenderer
, and the ClassBreakRenderer
. Each has its appropriate use cases.
The SimpleRenderer
is the simplest of the renderers because it only assigns one symbol. It accepts a default symbol, and assumes that you'll only insert graphics of the same type as the symbol. Like other renderers, if you assign a SimpleRenderer
to a GraphicsLayer
, you can add Graphic objects to the GraphicsLayer
without them having a symbol assigned. Let's look at a code snippet that shows how to create and apply a SimpleRenderer
to the map's graphics layer:
require(["esri/map", "esri/renderers/SimpleRenderer", "esri/symbols/SimpleLineSymbol", "esri/Color", "dojo/domReady!"], function (Map, SimpleRenderer, LineSymbol, Color) { … Var map = new Map("map", {basemap: "OSM"}); var symbol = new LineSymbol().setColor(new Color("#55aadd")); var renderer = new SimpleRenderer(symbol); … map.graphics.setRenderer(renderer); });
The SimpleRenderer
has been expanded since version 3.7 of the API. Symbols can be modified in different ways, based on a range of expected values in a field. For instance, you can vary the symbol's color between two colors by setting the colorInfo
property of the SimpleRenderer. You can also change the size of a point according to a field value by setting its proportionSymbolInfo
property. Finally, you can rotate a symbol by its field value by setting its rotationInfo
property.
Sometimes you want to display different symbols for your graphics based on one of their attributes. You can do that with a Unique Value Renderer. Specific unique values can be highlighted, while values not in the list can be assigned a default symbol.
The constructor of the Unique Value Renderer accepts a default symbol, and up to three different fields to compare values against. If more than one field is used, a field delimiter string is required. Unique values and the associated symbols can then be loaded as objects within the renderer's info parameter. The info object requires the following:
- A unique value (
value
) - A symbol associated with that value (
symbol
) - A symbol label (
label
) - A description of the unique value (
description
)
Sometimes you want to classify your graphics by where they fit in certain ranges of values, like population or Gross Domestic Product (GDP). The Class Break Renderer helps you to organize the graphics in a visual manner. This renderer looks at a particular field value in a graphic, and finds which class break range graphic's field value fits between. It then assigns the corresponding symbol to that graphic.
When constructing a Class Break Renderer, you assign a default symbol, and the field that will be evaluated to modify the symbol. Class breaks are assigned through an info property of the renderer. The info array accepts a JavaScript object containing the following:
- The minimum value of the class break (
minValue
) - The maximum value of the class break (
maxValue
) - The symbol (
symbol
) - A label describing the break (
label
) - A description of the class break (
description
)