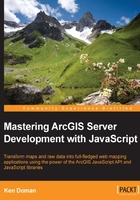
Drawing a map
When working with the ArcGIS JavaScript API, one of the first things you'll want to do is to create a map. The map transforms a simple div element into an interactive canvas where you can display, request, and even edit geographic data. The map is a complicated object, so we'll spend a significant amount of time focusing on it. Let's learn how to make a map in our scripts.
Constructor options
When a map is first created using the ArcGIS JavaScript API, the developer has numerous parameters that can be used to configure the map. We were exposed to some of those parameters in the previous chapter, when we created our first map application. Let's look into a few of them a little more closely.
The Autoresize function, which by default is true, tells the map whether it should resize itself when the browser changes size or orientation. If you have a full screen map application, and you maximize or resize your browser, the map will automatically adjust and fill the space assigned to it. The map will keep the same zoom level, but more or less only the edges of the map will be visible when you resize your browser.
Tip
If your application hides, moves, or animates your maps' appearance onto or off the screen, the autoresize functionality could lead to some strange behavior in the map. For example, if the map is made to slide in from the right, the mouse scroll and some zoom functionality may zoom to the center-left point, rather than the center of the map. With map animations on and off screen, it is best to set the autoresize to false.
The basemap is an optional string value that provides background imagery to lay your data on. ESRI serves a variety of background imagery, depending on how you want to frame your data. All of ESRI's basemaps are tiled images published in the Web Mercator projection (WKID 102100). For best performances, layers added on top of an ESRI basemap should be in the same projection. Otherwise, ArcGIS Server will have to reproject dynamic map services, and tiled map services may not show up if the spatial references do not match. You can see an example highlighted from the previous chapter in the following code snippet:
var map = new Map("map", {
basemap: "national-geographic",
center: [-95, 45],
zoom: 3
});
The background imagery options available from ESRI include:
street
: An ESRI street mapsatellite
: Satellite/Aerial photographyhybrid
: Combination of street map and satellitetopo
: Topographic map with elevation and contoursgray
: Light gray/minimalist backgroundoceans
: Oceans and bathymetry backgroundnational-geographic
: National Geographic-themedosm
: OpenStreetMap-themed
The center, extent, zoom, and scale parameters can be used to override the other map layer settings and set what area is displayed on the map. The center can be either a two number array containing the longitude and the latitude of the center point, or an ESRI point. The extent describes in map coordinates which edges of the map should be shown: right, left, top or bottom. If a tiled layer is present, the zoom instructs which level of display, from furthest out to furthest in, the map should show. The scale tells the map how many units of measure relate to one unit in reality (for example, a scale value of 500 means that 1 inch on the map represents 500 inches in real life).
When a map loads layers, the levels of details (LODs) are defined by the first tiled layer loaded in the map. You can override those LODs when creating a map, by passing an array of objects containing a level (number: 0, 1, 2, and so on), a scale (number: for 1:500 scale, you would use 500), and a corresponding resolution (number). This is helpful if you want to limit the user's ability to zoom way outside the view of your map, or if some of your tiled data has a finer resolution than your basemap will show.
Now that we've learned how adjust the parameters of a map object, let's look at its different parts.
Properties
The map object provides a number of properties that can be used in a mapping application. Some are read-only, and tell the status of the map. Others can be manipulated, and the changes can have significant impact on the map. While there are far more properties, we'll take a look at a few of them.
After you've created a map object, there are some things you can't do to the map until it has at least one layer loaded. When a map loads its first layer, it sets its loaded property to true. Then you can manipulate the map, pan around, search for layers, and modify its behavior.
A common programming error is not testing whether the map is loaded before doing something to it, like adding search result graphics from a query. If you have an extremely fast internet connection, and your ArcGIS Server is right next to you, the map might be loaded right after you add a layer. But, for internet users outside your server room, the map may not load that quickly. It's best to check the loaded property before continuing, as in the following example:
if (map.loaded) { doSomethingwithMap(map); } else { map.on("load", doSomethingwithMap); }
The map object keeps a list of references to the layers added to it. Map layer references are stored as an array of strings called layerIds
. The first layerId in the list refers to the bottom most layer in the stack, while the last id string in the list refers to the top layer visible on the map. This is handy if you need to search for a particular layer in the list, or if you need to do something to all the layers loaded in the map.
The map's spatialReference
property refers to the long list of mathematical equations used to represent a round earth on a flat map. This attribute is often (but not always) shared by the map and the layers and graphics contained within. We'll go into more depth studying spatial reference in the Geometry spatial reference section.
Methods
The map object has a number of useful methods, which are functions tied into the map object. These methods help you add items to the map, move around, zoom in and out, and manipulate map behavior. Let's take a look at some of them.
Layers can be added and removed from the map as needed. If you want to add one map layer at a time, you can call on the map's addLayer()
method. To add multiple layers at once, you have to put all those layers in an array and call the map's addLayers()
method. Map layers can be removed with the removeLayer()
method.
Retrieving layers is as simple as calling the map's getLayer()
method. The getLayer()
method is passed an id string for the layer. A list of the valid ids can be found in the map's layerIds
array, or in the map's GraphicsLayerId
property. If no layers are found that match the getLayer()
parameter, the method returns a null.
The map contains several methods for navigating the map. Some affect the location on the map, and others affect how zoomed in the user is. Here is a list of the most common methods used to manipulate your position on the map:
setZoom()
: This sets the map zoom level, moving further in or further out.setScale()
: Similar tosetZoom()
, but tries to change the map's scale to match the value passed.setLevel()
: Similar tosetZoom()
, but works only in maps with a tiled service. Based on the integer passed into the function, the map will zoom to the corresponding zoom level, if the map has tiled layers.setExtent()
: When passed a bounding box, this message will attempt to set the map's boundaries to match the bounding box. This affects both zoom level and position.centerAndZoom()
: Accepts a center point and a zoom level, then centers the map on that point and attempts to zoom to the zoom level. This affects both position and zoom level.centerAt()
: When this function accepts a point, it attempts to pan the map over so that the point is in the center of the map.
There are many map navigation actions that can be enabled or disabled using map methods. You can control what happens when the user clicks, double-clicks, or shift-double-clicks on the map. You can enable or disable panning with the mouse, as well as scrolling with the scroll wheel. You can even disable an enable-all navigation event for the map. There may be times you want to disable navigation. For instance, if your map is navigated by clicking on links, you may not want the user to wander too far on your map.
Remember that these settings cannot be modified until the map is loaded. But if the map hasn't loaded yet, how are we supposed to know when it loads? For an answer, we'll look into a feature common to most JavaScript libraries, events.
Events
Events are important when working with asynchronous programming in JavaScript. The map object has a number of events it fires before, during, and after it makes some important changes, or finishes certain tasks. These events can be listened to through the map's built-in on()
function. Let's look at some of the more common events you'll be working with:
load
: A map event that fires after the map has loaded its first layer.click
: This fires when the user clicks on the map. Among other event items, it returns the point the user clicked as amapPoint
property of the event object.extent-change
: This fires when the map either changes location, or changes zoom level. Both of these factor into calculating the map's extent.layer-add
: This fires when any new layer is added to the map.layers-add-result
: This fires after multiple layers are loaded using theaddLayers()
method.mouse-over
: This fires when the mouse is moved over the map.update-start
: This event fires before layers are loaded, either by adding new layers, or by moving around the map and triggering the map to download more map data.update-end
: This event fires after the map loads the data from its map services.