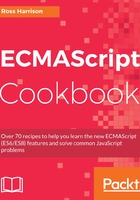
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open your command-line application and navigate to your workspace.
- Create a new folder named 3-08-simulating-finally.
- Copy or create an index.html that loads and runs a main function from main.js.
- Create a main.js file with a main function that logs out messages for before and after promise creation:
export function main() { console.log('Before promise created'); console.log('After promise created'); }
- Create a function named addBoosters that throws an error if its first parameter is false:
function addBoosters(shouldFail) { if (shouldFail) { throw new Error('Unable to add Boosters'); } return { boosters: [{ count: 2, fuelType: 'solid' }, { count: 1, fuelType: 'liquid' }] }; }
- Use Promise.resolve to pass a Boolean value that is true if a random number is greater than 0.5 to addBoosters:
export function main() { console.log('Before promise created'); Promise.resolve(Math.random() > 0.5)
.then(addBoosters) console.log('After promise created'); }
- Add a then function to the chain that logs a success message:
export function main() { console.log('Before promise created'); Promise.resolve(Math.random() > 0.5) .then(addBoosters) .then(() => console.log('Ready for launch:')) console.log('After promise created'); }
- Add a catch to the chain and log out the error if thrown:
export function main() { console.log('Before promise created'); Promise.resolve(Math.random() > 0.5) .then(addBoosters) .then(() => console.log('Ready for launch: ')) .catch(console.error) console.log('After promise created'); }
- Add a then after the catch, and log out that we need to make an announcement:
export function main() { console.log('Before promise created'); Promise.resolve(Math.random() > 0.5) .then(addBoosters) .then(() => console.log('Ready for launch: ')) .catch(console.error)
.then(() => console.log('Time to inform the press.')); console.log('After promise created'); }
- Start your Python web server and open the following link in your browser:
http://localhost:8000/. - If you are lucky and the boosters are added successfully, you'll see the following output:
- If you are unlucky, you'll see an error message like the following: