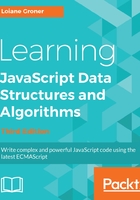
上QQ阅读APP看书,第一时间看更新
Iterator functions
Sometimes, we need to iterate the elements of an array. We have learned that we can use a loop construct to do this, such as the for statement, as we saw in some previous examples.
JavaScript also has some built-in iterator methods that we can use with arrays. For the examples in this section, we will need an array and a function. We will use an array with values from 1 to 15 and a function that returns true if the number is a multiple of 2 (even) and false otherwise. The code is presented as follows:
function isEven(x) { // returns true if x is a multiple of 2. console.log(x); return x % 2 === 0 ? true : false; } let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15];
return (x % 2 == 0) ? true : false can also be represented as return (x % 2 == 0).
To simplify our code, instead of declaring functions using the ES5 syntax, we will use the ES2015 (ES6) syntax as we learned in Chapter 2, ECMAScript and TypeScript Overview. We can rewrite the isEven function using arrow functions:
const isEven = x => x % 2 === 0;