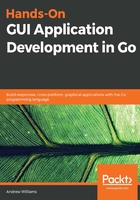
Menu
At the time of writing, andlabs UI doesn't expose a menu API (despite ui.NewWindow() taking a hasMenubar parameter). There is a project underway to properly expose menu functionality to the Go API, but for now it's only available if you work with the underlying libui C code. The menu defined in the C library can be accessed from a Go project by adding a little CGo code, such as the following:
/*
void onMenuNewClicked(uiMenuItem *item, uiWindow *w, void *data) {
void menuNewClicked(void);
menuNewClicked();
}
int onQuit(void *data) {
return 1;
}
void loadMenu() {
uiMenu *menu;
uiMenuItem *item;
menu = uiNewMenu("File");
item = uiMenuAppendItem(menu, "New");
uiMenuItemOnClicked(item, onMenuNewClicked, NULL);
uiMenuAppendSeparator(menu);
item = uiMenuAppendQuitItem(menu);
uiOnShouldQuit(onQuit, NULL);
menu = uiNewMenu("Help");
item = uiMenuAppendItem(menu, "About");
}
*/
import "C"
The code snippet sets up a click handler for a New menu item, and a quit handler for the Quit menu item (which is a special item due to macOS handling a quit menu item differently). Then we have a loadMenu() function, which sets up a File menu to which the child items are added, with a separator, and a currently-empty Help menu.
To compile this code correctly will require the cfuncs.go file knowing where the header file and C library are stored. Before running this code make sure that the CFLAGS and LDFLAGS show the correct locations. While the code to build a menu is not very complicated, the CGo configuration and linking is rather complex, and as such, may not be recommended:

The result should look similar to this screenshot, which was taken on a Linux computer:

There is a complete menu project in the code repository for this book. Unfortunately, it isn't a cross-platform project and may not execute correctly on every operating system or version of Go.